Ternary Operator in C
Learn via video course
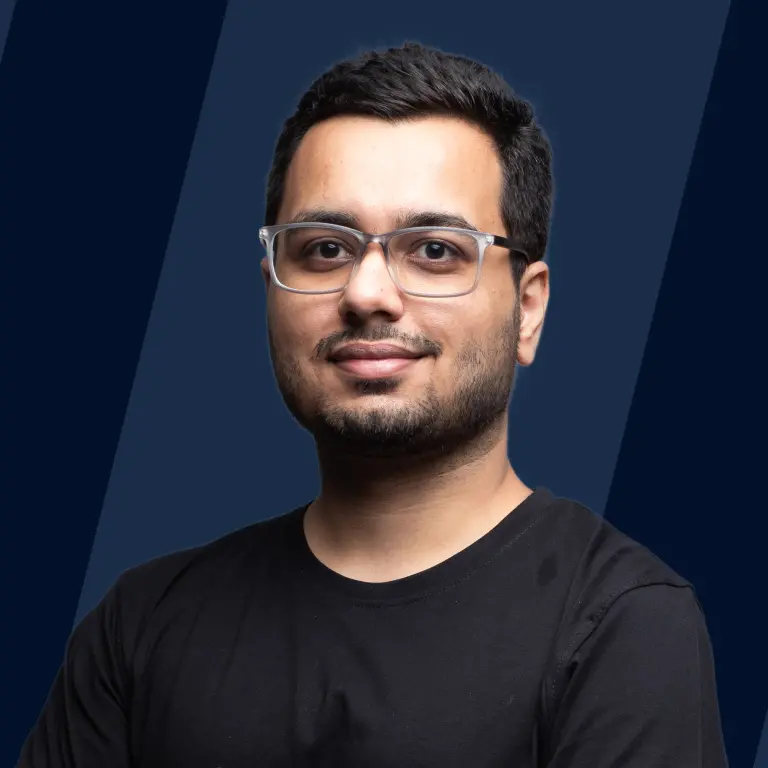
Overview
Ternary Operator in C is an operator which takes three operands or variables, unlike the other operators which take one or two operands. Ternary operator in C is also known as the Conditional Operator. It is a way to shorten the simple if-else code of the block.
Scope
- The article introduces you to the Ternary Operator in C with examples.
- The article contains some examples so you can easily learn about how to implement the ternary operator in C.
What is a Ternary Operator in C
Using the Ternary operator in c is a way to shorten the if-else code block in C/C++. So before you move further in this article, please go through the C if-else statement. (if you are a beginner).
Ternary Operator in C takes three arguments:
- The first argument in the Ternary Operator in C is the comparison condition.
- The second argument in the Ternary Operator in C is the result if the condition is true.
- The third argument in the Ternary Operator in C is the result if the condition is false.
So, according to the above three arguments in the ternary operator in c, we can say that the Ternary operator in C allows us to execute different code depending on the first argument, i.e. based on condition.
The symbol for the Ternary operator in C is ? :.
Syntax
The syntax of the Ternary Operator in C is:
Syntax:
Working of Syntax:
- If the condition in the ternary operator is met (true), then the exp2 executes.
- If the condition is false, then the exp3 executes.
Example:
The following example explains the working of the Ternary Operator in C.
So, if the condition 10 > 15 is true (which is false in this case) mxNumber is initialized with the value 10 otherwise with 15. As the condition is false so mxNumber will contain 15. This is how Ternary Operator in C works.
NOTE: Ternary operators in C, like if-else statements, can be nested.
Flow Chart for Ternary Operator in C
The flow chart of the Ternary Operator in C looks like this:
Let's understand this flow chart of Ternary Operator in C:-
Suppose we have taken a ternary operator statement exp1? exp2: exp3, if our exp1 met the condition or yield result in true the control flows to exp2. Similarly, if exp1 gives a false result, then our control goes to exp3.
Therefore, if the exp1, a condition that is true, then control flows to the True-Expression otherwise, control goes to the False_Expression. And if there is any next statement, the control goes to that statement, like in the above example variable mxNumber gets the value 15.
Isn't it similar to the simple if-else code in C ? YES !! That's why the Ternary Operator in C is also known as Conditional Operator as its works in the same way as if-else works in C.
Examples
These examples will teach us how to use the Ternary operator in C.
Example #1
Find the maximum number from the given two integer type numbers using the if-else block in C and with the Ternary Operator in C.
Using if-else block
Output:
In the above code, we have two integer type variables named num1 and num2 and they contain values 10 and 15 respectively. As we have to find out which one is the maximum number among these two variables. We applied a condition num1 >= num2, and according to the result of this condition, our mxNumber will contain the maximum number from these two numbers.
Using Ternary Operator in C
This example demonstrates how to utilize the Ternary Operator in C.
Output:
In the above code, we write down the previous code if-else condition in the form of the ternary operator in c. Using the ternary operator in C, we can easily shorten our code, which is memory efficient also. The working of the above code is the same as the previous example code. So it's totally up to you to either use a simple if-else block or Ternary Operator in c both ways are correct but try to use the ternary operator because it looks neat and clean with a memory-efficient advantage.
Example #2
Find out the given number is even or not using Ternary Operator in C.
Output:
In the above code, we are going to check given number is even or not using the ternary operator in c. To do the same, we applied the condition num%2 == 0 which checks our number and, based on the result, it's going to print the number is even or not.
Summary
- The ternary operator is used to execute a different piece of code based on the result of the condition.
- Ternary Operator is a way to shorten the if-else block of code in C.
- The symbol of the ternary operator in c is ?:
- In C, the ternary operator is used to minimize code size and improve compiler efficiency.
- The Ternary Operator in c is also called Conditional Operator.